[formatting strings in rust]
written 12/15/2024
format!("{}", your_mom)
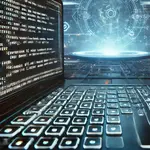
Formatting Strings
I asked Claude how to format strings in Rust. It introduced me to the format!
macro.
Here is what Claude had to say.
In Rust, there are several ways to format strings. I'll show you the most common methods:
- Using
format!
Macro
1let name = "Alice";
2let age = 30;
3let formatted = format!("My name is {} and I am {} years old", name, age);
4println!("{}", formatted);
- Using Positional Arguments
1let formatted = format!("First: {0}, Second: {1}, First again: {0}", "hello", "world");
- Named Parameters
1let formatted = format!("Name: {name}, Age: {age}", name="Bob", age=25);
- Specifying Formatting Traits
1// Debugging format
2let debug_str = format!("Debug: {:?}", vec![1, 2, 3]);
3
4// Precision for floating-point numbers
5let pi = 3.14159;
6let precise = format!("Pi to two decimal places: {:.2}", pi);
- Padding and Alignment
1let padded = format!("{:10}", "hi"); // Right-aligned, width 10
2let left_aligned = format!("{:<10}", "hi"); // Left-aligned, width 10
3let zero_padded = format!("{:0>5}", 42); // Zero-padded to width 5
The format!
macro is versatile and allows you to create formatted strings without printing them, while println!
directly prints to the console. The syntax is consistent across these methods, making string formatting in Rust quite flexible and powerful.