[random numbers in rust]
written 12/15/2024
for i in range 0..?
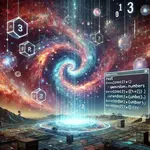
Generating Random Numbers
I asked Calude how to generate random numbers in Rust. Here is the response:
I'll show you how to generate random numbers in Rust using the rand crate, which is the most common way to generate random numbers in Rust.
First, you'll need to add the rand crate to your Cargo.toml file:
1[dependencies]
2rand = "0.8.5"
Here are different ways to generate random numbers:
- Generating a Random Integer
1use rand::Rng;
2
3fn main() {
4 let mut rng = rand::thread_rng();
5
6 // Random integer between 1 and 10
7 let random_number = rng.gen_range(1..=10);
8 println!("Random number: {}", random_number);
9}
- Generating a Random Float
1use rand::Rng;
2
3fn main() {
4 let mut rng = rand::thread_rng();
5
6 // Random float between 0.0 and 1.0
7 let random_float = rng.gen::<f64>();
8 println!("Random float: {}", random_float);
9
10 // Random float in a specific range
11 let random_range_float = rng.gen_range(0.0..10.0);
12 println!("Random float in range: {}", random_range_float);
13}
- Random Boolean
1use rand::Rng;
2
3fn main() {
4 let mut rng = rand::thread_rng();
5
6 let random_bool = rng.gen_bool(0.5); // 50% true, 50% false
7 println!("Random boolean: {}", random_bool);
8}
- Random Choice from a Collection
1use rand::seq::SliceRandom;
2
3fn main() {
4 let mut rng = rand::thread_rng();
5
6 let choices = vec![1, 2, 3, 4, 5];
7
8 // Random element
9 if let Some(chosen) = choices.choose(&mut rng) {
10 println!("Randomly chosen: {}", chosen);
11 }
12
13 // Shuffling a vector
14 let mut nums = vec![1, 2, 3, 4, 5];
15 nums.shuffle(&mut rng);
16 println!("Shuffled vector: {:?}", nums);
17}
- Random Distribution (Noraml/Gaussian)
1use rand::distributions::{Normal, Distribution};
2
3fn main() {
4 let mut rng = rand::thread_rng();
5
6 // Normal distribution with mean 0.0 and standard deviation 1.0
7 let normal = Normal::new(0.0, 1.0).unwrap();
8 let random_gaussian = normal.sample(&mut rng);
9 println!("Gaussian distributed number: {}", random_gaussian);
10}
Key points to remember:
- rand::thread_rng() creates a random number generator local to the current thread
- gen_range() lets you specify a range (inclusive or exclusive)
- gen() generates a random number of the specified type
- Always import rand::Rng to use these methods
Each time you run the program, you'll get different random numbers. The rand crate provides a robust and secure way to generate random numbers in Rust.